-----
iOS Programming Basic: How Does the Hello World App Work?
I hope you enjoy the first iOS programming tutorial and already created your first app. Before we move onto the next tutorial and build a more complex app, let’s step back and have a closer look at the Hello World app. It’ll be good for you to understand some of the Objective-C syntax and the inner workings of the app.
So far you follow the step-by-step guide to build the Hello World app. But as you go through the tutorial, you may come across these questions:
- What are those .xib, .h and .m file?
- What are those “ugly” code inside “showMessage”? What do they mean?!
- What actually happens after you taps the “Hello World” button? How does the button trigger the “showMessage” action?
- How does the “Run” button in Xcode work?
I want you to focus on exploring the Xcode environment so I didn’t explain any of the above in the previous post. Yet it’s essential for every developer to understand the inner details behind the code and grasp the basic concept of iOS programming. For some technical concepts, they may be a bit hard to understand particularly you have no programming background. Don’t worry, however. This is just a start. As you move on and write more code in later tutorials, you’ll get better understanding about iOS programming. Just try your best to learn as much as possible.
Interface Builder, Header and Implementation Files
First, what are those .xib, .h and .m files? This is a very good question raised by one of the readers. Under the Project Navigator, you should find three main types of files – .xib, .h and .m. (If you expand the “Supporting Files” folder, you’ll find other file types such as plist and framework. But for now, let’s forget about them first. We’ll talk about them later.)
.xib – For files with .xib extension, they’re Interface Builder files that store the application’s user interface (UI). As you click on the .xib file, Xcode automatically switches to the Interface Builder for you to edit the UI of the app via drag-and-drop.

Interface Builder in Xcode
.h and .m – Files with .h extension refers to the header files while those with .m extension are the implementation files. Like most of the programming languages, the source code of Objective-C is divided into two parts: interface and implementation.
Well, to put in analogy that you can better understand both terms, let’s consider a TV remote. It’s convenient to control the volume of a TV set wirelessly with a remote. To increase the speaker volume, you press the “Volume +” button. To switch channel, you simply key in the channel number. Let me ask you. Do you know what happens behind the scene when pressing the “Volume” button? Probably not. I believe most of us don’t know how the remote communicates with the TV set and controls the speaker volume. What we just know is, that button is used for changing the volume. In this example, the button that interacts with you is the “interface” and the inner detail which is hidden behind the button is referred as the “implementation”.
Now you should have a better idea about interface and implementation. Let’s go back to the code. In Objective-C, interfaces of a class are organized in “.h” file. We use the syntax “@interface” to declare the interface of a class. Take a look at the HelloWorldViewController.h, which is the header file:
1
2 3 4 5 |
@interface HelloWorldViewController : UIViewController
-(IBAction)showMessage; @end |
It starts with “@interface” followed by HelloWorldViewController, which is the class name. Inside, it declares a “showMessage” action, which is known as a method call.
Like the “Volume” button, apparently we do not know how the “showMessage” action works. You just know it’s used to display a message on screen. The actual implementation is put in the HelloWorldViewController.m, the implementation file:
1
2 3 4 5 6 7 8 9 10 11 12 13 14 |
@implementation HelloWorldViewController
// I've removed other methods for better reading. Focus on the showMessage method first. - (IBAction)showMessage { UIAlertView *helloWorldAlert = [[UIAlertView alloc] initWithTitle:@"My First App" message:@"Hello, World!" delegate:nil cancelButtonTitle:@"OK" otherButtonTitles:nil]; // Display the Hello World Message [helloWorldAlert show]; } @end |
As you can see from the above, you use the declaration “@implementation” to declare an implementation. Inside the “showMessage”, it’s the actual code defined to display the alert message on screen. You may not understand every single line of code inside the “showMessage” method. In brief, it creates an UIAlertView with “My First App” as the title and “Hello, World” as the message. It then calls up the “show” method and request iOS to display the pop-up message on screen.
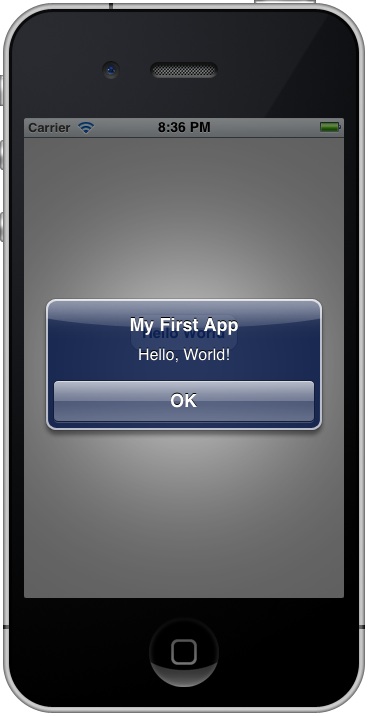
Hello World App
It’s vital for you to understand the concept of interface and implementation. If you have any question, feel free to raise your question at our forum.
Behind the Touch and Tap
What actually happened after tapping the “Hello World” button? How does the “Hello World” button invoke the “showMessage” method to display the “Hello World” message?
Recalled that you established a connection between the “Hello World” button and the “sendMessage” action in Interface Builder. Try opening up the “HelloWorldViewController.xib” again and select the “Hello World” button. Click the “Sent Events” button in the Utility area to open the Sent Events.
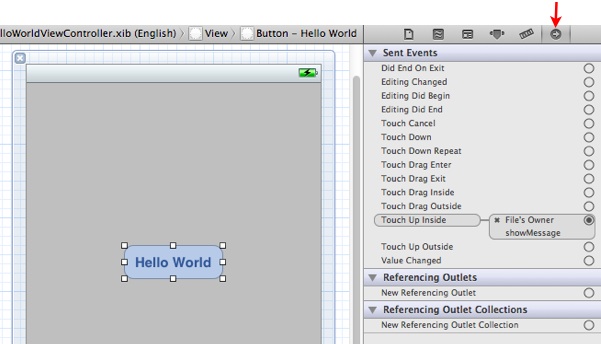
The Sent Events section shows you all connections between events and actions. As you can see in the above figure, the “Touch Up Inside” event is connected to the “showMessage” action. In iOS, apps are event-driven. The control/object (e.g. UIButton) listens for certain events (e.g. touches and taps). When the event is triggered, the object calls up the preset action that associates with the event.
In our Hello World app, when users lift up the finger inside the button, the “Touch Up Inside” event is triggered. Consequently, it calls up the “showMessage” action to display the “Hello World” message.
The below illustration sums up the event flow and what I have just described.

Event and Message Flow of Hello World App
Behind the Scene of the “Run” Button
When you click the “Run” button, Xcode automatically launches the Simulator and runs your app. But what happens behind the scene? As a programmer, you have to look into the entire process.

The entire process can be broken into three phases: compile, package and run.
Compile – You probably think iOS understands Objective-C code. In reality, iOS only reads machine code. The Objective-C code is only for you, the programmer to write and read. To make iOS understand the source code of the app, we have to go through a translation process to translate the Objective-C code into machine code. This process is referred as “compile”. Xcode already comes with a built-in compiler to compile the source code.
Package – Other than source code, an app usually contains resource files such as images, text files, xib files, etc. All these resources are packaged to make up the final app.
We used to refer these two processes as the “build” process.
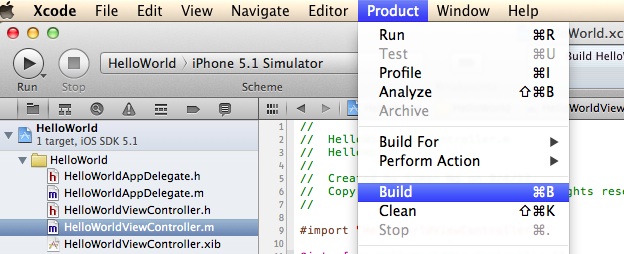
Run – This actually launches the Simulator and loads your app.
No comments:
Post a Comment